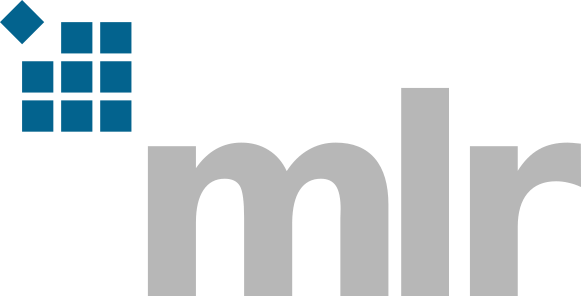
Feature Selection with Random Search
Source:R/FSelectorBatchRandomSearch.R
mlr_fselectors_random_search.Rd
Feature selection using Random Search Algorithm.
Source
Bergstra J, Bengio Y (2012). “Random Search for Hyper-Parameter Optimization.” Journal of Machine Learning Research, 13(10), 281–305. https://jmlr.csail.mit.edu/papers/v13/bergstra12a.html.
Details
The feature sets are randomly drawn.
The sets are evaluated in batches of size batch_size
.
Larger batches mean we can parallelize more, smaller batches imply a more fine-grained checking of termination criteria.
Control Parameters
max_features
integer(1)
Maximum number of features. By default, number of features in mlr3::Task.batch_size
integer(1)
Maximum number of feature sets to try in a batch.
Super classes
mlr3fselect::FSelector
-> mlr3fselect::FSelectorBatch
-> FSelectorBatchRandomSearch
Examples
# Feature Selection
# \donttest{
# retrieve task and load learner
task = tsk("penguins")
learner = lrn("classif.rpart")
# run feature selection on the Palmer Penguins data set
instance = fselect(
fselector = fs("random_search"),
task = task,
learner = learner,
resampling = rsmp("holdout"),
measure = msr("classif.ce"),
term_evals = 10
)
# best performing feature subset
instance$result
#> bill_depth bill_length body_mass flipper_length island sex year
#> <lgcl> <lgcl> <lgcl> <lgcl> <lgcl> <lgcl> <lgcl>
#> 1: TRUE TRUE TRUE FALSE FALSE FALSE TRUE
#> features n_features classif.ce
#> <list> <int> <num>
#> 1: bill_depth,bill_length,body_mass,year 4 0.06956522
# all evaluated feature subsets
as.data.table(instance$archive)
#> bill_depth bill_length body_mass flipper_length island sex year
#> <lgcl> <lgcl> <lgcl> <lgcl> <lgcl> <lgcl> <lgcl>
#> 1: FALSE TRUE FALSE TRUE TRUE TRUE FALSE
#> 2: TRUE TRUE TRUE TRUE TRUE TRUE FALSE
#> 3: TRUE TRUE TRUE TRUE TRUE FALSE TRUE
#> 4: FALSE FALSE TRUE FALSE TRUE FALSE FALSE
#> 5: FALSE TRUE FALSE TRUE FALSE FALSE FALSE
#> 6: TRUE TRUE TRUE FALSE FALSE FALSE TRUE
#> 7: TRUE FALSE FALSE FALSE FALSE FALSE FALSE
#> 8: FALSE FALSE FALSE FALSE TRUE FALSE FALSE
#> 9: TRUE FALSE FALSE FALSE FALSE FALSE FALSE
#> 10: TRUE FALSE FALSE FALSE FALSE TRUE FALSE
#> classif.ce runtime_learners timestamp batch_nr warnings errors
#> <num> <num> <POSc> <int> <int> <int>
#> 1: 0.09565217 0.006 2025-01-16 10:17:54 1 0 0
#> 2: 0.09565217 0.006 2025-01-16 10:17:54 1 0 0
#> 3: 0.09565217 0.005 2025-01-16 10:17:54 1 0 0
#> 4: 0.26086957 0.006 2025-01-16 10:17:54 1 0 0
#> 5: 0.10434783 0.005 2025-01-16 10:17:54 1 0 0
#> 6: 0.06956522 0.006 2025-01-16 10:17:54 1 0 0
#> 7: 0.20000000 0.005 2025-01-16 10:17:54 1 0 0
#> 8: 0.34782609 0.007 2025-01-16 10:17:54 1 0 0
#> 9: 0.20000000 0.006 2025-01-16 10:17:54 1 0 0
#> 10: 0.20000000 0.006 2025-01-16 10:17:54 1 0 0
#> features n_features
#> <list> <list>
#> 1: bill_length,flipper_length,island,sex 4
#> 2: bill_depth,bill_length,body_mass,flipper_length,island,sex 6
#> 3: bill_depth,bill_length,body_mass,flipper_length,island,year 6
#> 4: body_mass,island 2
#> 5: bill_length,flipper_length 2
#> 6: bill_depth,bill_length,body_mass,year 4
#> 7: bill_depth 1
#> 8: island 1
#> 9: bill_depth 1
#> 10: bill_depth,sex 2
#> resample_result
#> <list>
#> 1: <ResampleResult>
#> 2: <ResampleResult>
#> 3: <ResampleResult>
#> 4: <ResampleResult>
#> 5: <ResampleResult>
#> 6: <ResampleResult>
#> 7: <ResampleResult>
#> 8: <ResampleResult>
#> 9: <ResampleResult>
#> 10: <ResampleResult>
# subset the task and fit the final model
task$select(instance$result_feature_set)
learner$train(task)
# }